Python – How to use the Stablediffusion API in 4 easy steps
Step 1 – Generate a bearer token
Login and then create an application from the My account menu.
Generate a bearer token from your new application.
Step 2 – Start a new thread with Stablediffusion
You may select an ai_version_code and an engine. For example : stablediffusion_text2img and stable-diffusion-xl-1024-v0-9.
Send an http POST request to create a new thread.
import requests import time bearer = '<<your_token_here>>' # CREATE THREAD # ------------- url = "https://app.ai-client.com/api/v1/threads" payload={'ai_version_code': 'stablediffusion_text2img'} headers = { 'Accept': 'application/json', 'Authorization': 'Bearer ' + bearer } response = requests.request("POST", url, headers=headers, data=payload) thread = response.json()['data'] print(thread)
This will return :
{ "guid":"44142638-4934-4bf5-9be7-b4b5a6368f44", "ai_code":"stablediffusion", "ai_version_code":"stablediffusion_text2img", "title":"New thread with Stable Diffusion Image Generation", "created_at":"2023-07-13T19:56:25.000000Z", "updated_at":"2023-07-13T19:56:25.000000Z" }
Step 3 – Create a thread entry to send a message
You may use the thread object to create a new entry with a POST request.
url = "https://app.ai-client.com/api/v1/threads/" + thread['guid'] + "/entry" payload={ 'type': 'request', 'engine' : 'stable-diffusion-xl-1024-v0-9', 'resolution' : '1024x1024', # positive prompt: what we want to see 'prompts[0][text]' : "Delorean hacking time", 'prompts[0][weight]' : 1, # negative prompt: what we want to avoid 'prompts[1][text]' : "Clock", 'prompts[1][weight]' : -1, 'images' : 2, 'steps' : 45, 'style' : "neon-punk" } headers = { 'Accept': 'application/json', 'Authorization': 'Bearer ' + bearer } response = requests.request("POST", url, headers=headers, data=payload) thread_entry = response.json()['data'] print(thread_entry)
This will return :
{ "guid":"c7c088d3-7644-4385-b77c-a3ee98c4eed2", "thread_guid":"44142638-4934-4bf5-9be7-b4b5a6368f44", "type":"request", "error":"None", "content":{ "prompts":[ { "text":"Delorean hacking time", "weight":1 }, { "text":"Clock", "weight":-1 } ], "engine":"stable-diffusion-xl-1024-v0-9", "style":"neon-punk", "images":2, "steps":45, "resolution":"1024x1024" }, "waiting_response":true, "credits":0, "created_at":"2023-07-13T19:56:25.000000Z" }
Step 4 – Wait for a response from Stablediffusion
You must now wait for the AI’s response. The “waiting_response” property of your thread entry informs you of Stablediffusion’s response.
You may use the thread object to get all the thread entries. Send a GET request. You will see your thread entry of type “request” and the Stablediffusion thread entry of type “response“.
url = "https://app.ai-client.com/api/v1/threads/" + thread['guid'] + "/entries" headers = { 'Accept': 'application/json', 'Authorization': 'Bearer ' + bearer } response = requests.request("GET", url, headers=headers) thread_entries = response.json()['data']
This will return :
[ { "guid":"c7c088d3-7644-4385-b77c-a3ee98c4eed2", "thread_guid":"44142638-4934-4bf5-9be7-b4b5a6368f44", "type":"request", "error":"None", "content":{ "prompts":[ { "text":"Delorean hacking time", "weight":1 }, { "text":"Clock", "weight":-1 } ], "engine":"stable-diffusion-xl-1024-v0-9", "style":"neon-punk", "images":2, "steps":45, "resolution":"1024x1024" }, "waiting_response":false, "credits":0, "created_at":"2023-07-13T19:56:25.000000Z" }, { "guid":"a9e17dcc-a162-41ed-a1b4-94ecc40cdf90", "thread_guid":"44142638-4934-4bf5-9be7-b4b5a6368f44", "type":"response", "error":"None", "content":[ { "url":"https://app.ai-client.com/storage/stablediffusion_text2img/2212c664-7d5b-4fe5-b240-1f5e46e2e70e.png", "thumbnail_url":"https://app.ai-client.com/storage/stablediffusion_text2img/2212c664-7d5b-4fe5-b240-1f5e46e2e70e.png.thumbnail.jpg", "dimensions":"1024x1024", "filesize":1409734 }, { "url":"https://app.ai-client.com/storage/stablediffusion_text2img/e65893b4-acfb-459d-9ee4-796d724b9689.png", "thumbnail_url":"https://app.ai-client.com/storage/stablediffusion_text2img/e65893b4-acfb-459d-9ee4-796d724b9689.png.thumbnail.jpg", "dimensions":"1024x1024", "filesize":1685147 } ], "waiting_response":false, "credits":10, "created_at":"2023-07-13T19:57:09.000000Z" } ]
Full code example
import requests import time bearer = '<<your_token_here>>' # CREATE THREAD # ------------- url = "https://app.ai-client.com/api/v1/threads" payload={'ai_version_code': 'stablediffusion_text2img'} headers = { 'Accept': 'application/json', 'Authorization': 'Bearer ' + bearer } response = requests.request("POST", url, headers=headers, data=payload) thread = response.json()['data'] print(thread) # {'guid': '44142638-4934-4bf5-9be7-b4b5a6368f44', 'ai_code': 'stablediffusion', 'ai_version_code': 'stablediffusion_text2img', 'title': 'New thread with Stable Diffusion Image Generation', 'created_at': '2023-07-13T19:56:25.000000Z', 'updated_at': '2023-07-13T19:56:25.000000Z'} # CREATE THREAD ENTRY OF TYPE request # ----------------------------------- url = "https://app.ai-client.com/api/v1/threads/" + thread['guid'] + "/entry" payload={ 'type': 'request', 'engine' : 'stable-diffusion-xl-1024-v0-9', 'resolution' : '1024x1024', # positive prompt: what we want to see 'prompts[0][text]' : "Delorean hacking time", 'prompts[0][weight]' : 1, # negative prompt: what we want to avoid 'prompts[1][text]' : "Clock", 'prompts[1][weight]' : -1, 'images' : 2, 'steps' : 45, 'style' : "neon-punk" } headers = { 'Accept': 'application/json', 'Authorization': 'Bearer ' + bearer } response = requests.request("POST", url, headers=headers, data=payload) thread_entry = response.json()['data'] print(thread_entry) # {'guid': 'c7c088d3-7644-4385-b77c-a3ee98c4eed2', 'thread_guid': '44142638-4934-4bf5-9be7-b4b5a6368f44', 'type': 'request', 'error': None, 'content': {'prompts': [{'text': 'Delorean hacking time', 'weight': 1}, {'text': 'Clock', 'weight': -1}], 'engine': 'stable-diffusion-xl-1024-v0-9', 'style': 'neon-punk', 'images': 2, 'steps': 45, 'resolution': '1024x1024'}, 'waiting_response': True, 'credits': 0, 'created_at': '2023-07-13T19:56:25.000000Z'} # WAIT FOR 2 MINUTES # ------------------ time.sleep(120) # GET THREAD ENTRIES # ------------------ url = "https://app.ai-client.com/api/v1/threads/" + thread['guid'] + "/entries" headers = { 'Accept': 'application/json', 'Authorization': 'Bearer ' + bearer } response = requests.request("GET", url, headers=headers) thread_entries = response.json()['data'] print(thread_entries) # [{'guid': 'c7c088d3-7644-4385-b77c-a3ee98c4eed2', 'thread_guid': '44142638-4934-4bf5-9be7-b4b5a6368f44', 'type': 'request', 'error': None, 'content': {'prompts': [{'text': 'Delorean hacking time', 'weight': 1}, {'text': 'Clock', 'weight': -1}], 'engine': 'stable-diffusion-xl-1024-v0-9', 'style': 'neon-punk', 'images': 2, 'steps': 45, 'resolution': '1024x1024'}, 'waiting_response': False, 'credits': 0, 'created_at': '2023-07-13T19:56:25.000000Z'}, {'guid': 'a9e17dcc-a162-41ed-a1b4-94ecc40cdf90', 'thread_guid': '44142638-4934-4bf5-9be7-b4b5a6368f44', 'type': 'response', 'error': None, 'content': [{'url': 'https://app.ai-client.com/storage/stablediffusion_text2img/2212c664-7d5b-4fe5-b240-1f5e46e2e70e.png', 'thumbnail_url': 'https://app.ai-client.com/storage/stablediffusion_text2img/2212c664-7d5b-4fe5-b240-1f5e46e2e70e.png.thumbnail.jpg', 'dimensions': '1024x1024', 'filesize': 1409734}, {'url': 'https://app.ai-client.com/storage/stablediffusion_text2img/e65893b4-acfb-459d-9ee4-796d724b9689.png', 'thumbnail_url': 'https://app.ai-client.com/storage/stablediffusion_text2img/e65893b4-acfb-459d-9ee4-796d724b9689.png.thumbnail.jpg', 'dimensions': '1024x1024', 'filesize': 1685147}], 'waiting_response': False, 'credits': 10, 'created_at': '2023-07-13T19:57:09.000000Z'}]
See our Swagger API documentation for more information.
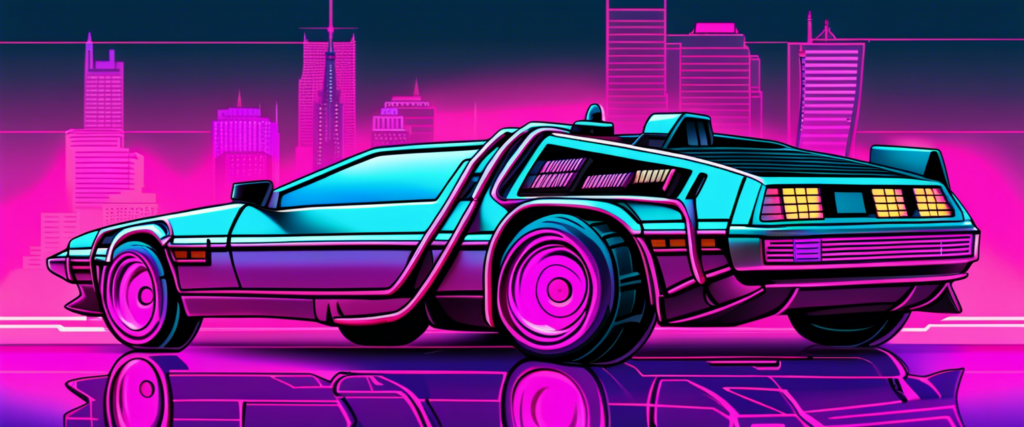